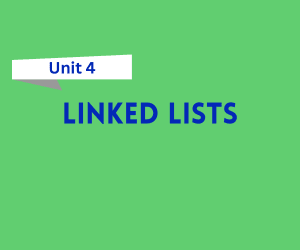
Free Download Linked lists Notes in pdf – Bca 3rd Semester. High quality, well-structured and Standard Notes that are easy to remember.
Click on the Download Button 👇
Linked Lists: Description, Key Points, and Features
A linked list is a fundamental data structure in computer science used for organizing and storing data elements. Unlike arrays, where elements are stored in contiguous memory locations, a linked list stores elements (known as nodes) that are scattered across memory and connected via pointers. This dynamic memory allocation provides flexibility for data manipulation.
Description of Linked Lists
A linked list is a linear data structure where each element (node) contains two components:
- Data: Stores the actual value or information.
- Pointer/Reference: Stores the memory address (reference) of the next node in the sequence.
The first node in a linked list is called the head, and the last node, which points to null (indicating the end of the list), is called the tail. A linked list can grow or shrink dynamically as elements are inserted or deleted.
Types of Linked Lists:
- Singly Linked List: Each node points to the next node in the sequence.
- Doubly Linked List: Each node contains two pointers, one pointing to the next node and the other to the previous node.
- Circular Linked List: The last node in the list points back to the head, forming a circular structure.
Key Operations in Linked Lists
Insertion: A new node can be added to the linked list at any position (beginning, middle, or end). Inserting at the head is an O(1) operation, while inserting in the middle or end may take O(n) time due to traversal.
Deletion: A node can be deleted from any position in the linked list. Similar to insertion, deleting the head is O(1), but deleting a node in the middle or end requires traversal.
Traversal: To access or display all elements, the list is traversed from the head to the tail.
Searching: Finding a node with a specific value requires traversing the list from the head to the node with the desired data. This can take O(n) time.
Key Points of Linked Lists
Dynamic Memory Allocation: Linked lists do not need a predefined size, making them suitable for situations where the number of elements may change frequently.
Efficient Insertions/Deletions: Inserting or deleting an element at the beginning of the list is a constant-time operation (O(1)). However, operations in the middle or at the end require traversal, making them O(n).
Memory Usage: Each node in a linked list requires extra memory for storing pointers, which increases the overall memory usage compared to arrays.
Non-Contiguous Memory: Linked lists store nodes in non-contiguous memory locations, making them more memory efficient in cases where memory fragmentation is an issue.
Flexible: Linked lists are highly flexible and can easily grow and shrink. This flexibility makes them useful for dynamic data structures like stacks, queues, and graphs.
Features of Linked Lists
Ease of Modification: Linked lists allow easy insertion and deletion of nodes, especially at the beginning. This is more efficient than arrays, where shifting elements is often required.
No Fixed Size: Linked lists do not require an initial size to be declared, unlike arrays. This makes them useful in scenarios where the amount of data is not known in advance or can vary significantly.
Efficient Use of Memory: As elements are added or removed, memory is allocated or deallocated dynamically, making efficient use of system memory. Memory wastage is minimized compared to arrays, which can leave empty slots after resizing.
Pointer-Based: Each node in a linked list contains pointers to the next node (or previous node in the case of a doubly linked list). This pointer-based structure enables dynamic growth but also requires careful management to avoid memory leaks and dangling pointers.
Variations:
- Singly Linked List: A node only points to the next node, providing simple, linear connections.
- Doubly Linked List: A node points to both the previous and next node, allowing traversal in both directions.
- Circular Linked List: The tail node points back to the head, forming a closed loop.
Advantages of Linked Lists
Efficient Memory Utilization: Unlike arrays, linked lists allocate memory as needed. No pre-allocation of memory is necessary.
Dynamic Sizing: Linked lists can expand or contract dynamically without wasting memory. This is a significant advantage in scenarios where the number of elements is unpredictable.
Ease of Insertion/Deletion: Inserting or deleting nodes is more efficient than arrays, especially for operations at the beginning or end.
Supports Various Data Structures: Linked lists can be used to implement stacks, queues, and other dynamic data structures, making them versatile in application.
Disadvantages of Linked Lists
Extra Memory Usage: The additional memory used for pointers increases the space complexity compared to arrays.
Slow Access Time: Accessing an element in a linked list requires traversal from the head node, leading to O(n) time complexity, whereas arrays allow direct access in O(1) time.
Complex Pointer Management: The use of pointers increases the complexity of the code and the risk of pointer-related errors such as memory leaks and null pointer exceptions.