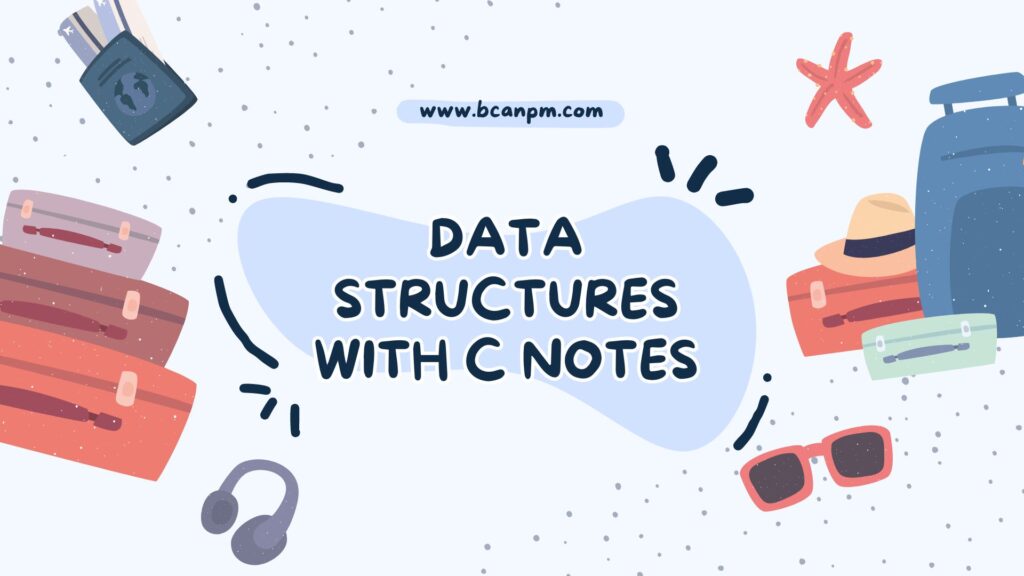
Free Download Data Structures with C Notes in pdf – Bca 3rd Semester. High quality, well-structured and Standard Notes that are easy to remember.
Welcome to Bcanpm.com
Bcanpm provides standard or well-structured Bca Notes for students. The notes are free to download. Each semester notes of Bca are available on www.bcanpm.com. In this post you can download notes of Data Structures with C (C 5). All units are available to download for free.
Data Structures with C Notes Unit 1 – 7
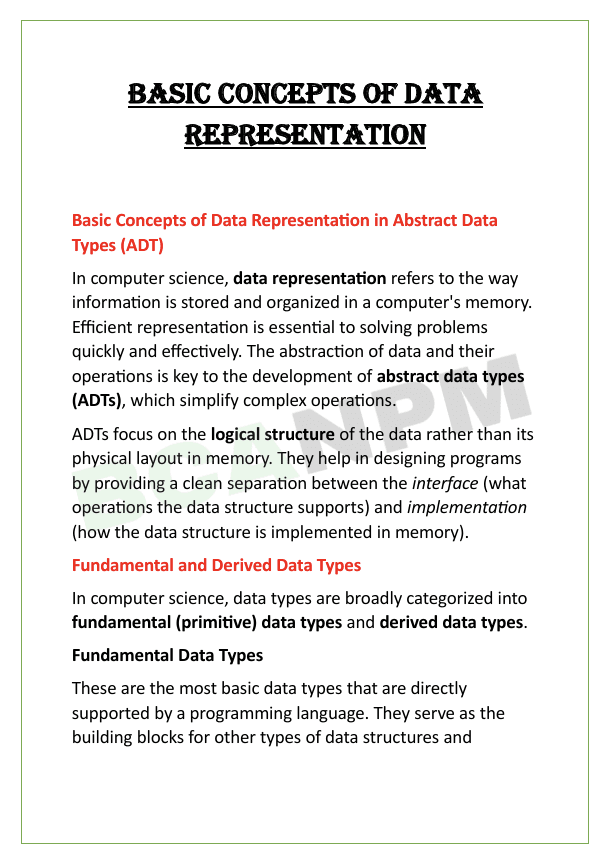
Unit 1: Basic concepts of data representation
“Data representation” refers to the methods used to encode and store data in a format that can be processed by computers.
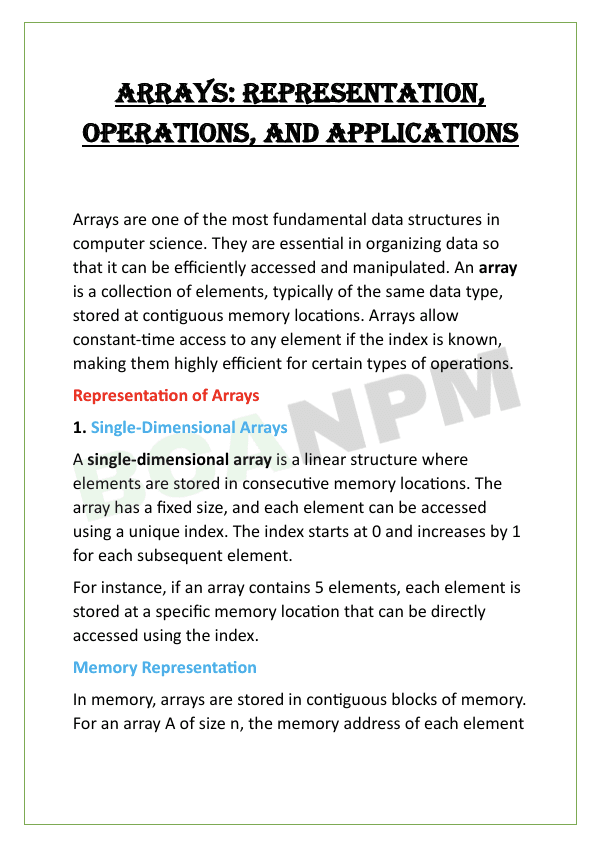
Unit 2: Arrays
An “Arrays” is a fundamental data structure used to store a collection of elements, typically of the same data type, in a contiguous block of memory.

Unit 3: Stacks and queues
“Stacks and queues” are both linear data structures used to manage collections of elements, but they differ in how elements are added and removed.

Unit 4: Linked lists
A “Linked lists” is a linear data structure in which elements, known as nodes, are stored in a sequence. Each node contains two parts: the data and a reference (or pointer) to the next node in the sequence.

Unit 5: Trees
A “Tree” is a hierarchical data structure composed of nodes, where each node stores a value and references to its child nodes.
Syllabus of Data Structures
UNIT – 1
1. Introduction to Data Structures
- Definition and Importance: Understanding the role of data structures in problem-solving and efficient algorithm design.
- Classification: Primitive and non-primitive data structures, linear and non-linear data structures.
UNIT – 2
2. Arrays
- Introduction to Arrays: Definition, types (one-dimensional, two-dimensional, multi-dimensional).
- Array Operations: Insertion, deletion, traversal, searching, and sorting.
- Applications: Practical applications of arrays in various scenarios.
UNIT – 3
3. Linked Lists
- Introduction to Linked Lists: Definition, advantages over arrays, types (singly, doubly, circular linked lists).
- Operations on Linked Lists: Insertion, deletion, traversal, searching, and reversing.
- Applications: Implementing stacks and queues using linked lists.
UNIT – 4
4. Stacks
- Definition and Concepts: LIFO (Last In, First Out) principle, stack operations (push, pop, peek).
- Implementation: Using arrays and linked lists.
- Applications: Function calls, expression evaluation (infix, postfix, prefix), recursion.
UNIT – 5
5. Queues
- Definition and Concepts: FIFO (First In, First Out) principle, queue operations (enqueue, dequeue, front, rear).
- Types of Queues: Simple queue, circular queue, priority queue, double-ended queue (deque).
- Implementation: Using arrays and linked lists.
- Applications: Job scheduling, simulation, breadth-first search (BFS).
UNIT – 6
6. Trees
- Introduction to Trees: Basic terminology (root, leaf, child, parent, sibling), types of trees (binary tree, binary search tree, AVL tree, etc.).
- Binary Trees: Representation, traversal methods (in-order, pre-order, post-order).
- Binary Search Trees (BST): Properties, operations (insertion, deletion, searching).
- Balanced Trees: AVL trees, rotations for balancing.
UNIT – 7
7. Graphs
- Introduction to Graphs: Basic terminology (vertices, edges, degree, path, cycle), types of graphs (directed, undirected, weighted, unweighted).
- Graph Representations: Adjacency matrix, adjacency list.
- Graph Traversals: Depth-first search (DFS), breadth-first search (BFS).
- Applications: Shortest path algorithms (Dijkstra’s, Floyd-Warshall), minimum spanning tree (Kruskal’s, Prim’s).
UNIT – 8
8. Hashing
- Introduction to Hashing: Hash functions, properties of good hash functions.
- Collision Resolution Techniques: Open addressing, chaining.
- Applications: Implementing dictionaries, databases.
UNIT – 9
9. Sorting and Searching Algorithms
- Sorting Algorithms: Bubble sort, selection sort, insertion sort, merge sort, quick sort, heap sort.
- Searching Algorithms: Linear search, binary search.
- Algorithm Analysis: Time complexity and space complexity.
UNIT – 10
10. File Handling
- Basic Concepts: Types of files (text, binary), file operations (open, close, read, write).
- File Handling in C: Using file pointers, seek, tell, read, write functions.
- Applications: Reading and writing data structures to files.